The objective of this project is to design a traffic light control system. This traffic light controller is used at the intersection that consists of a main road and two side roads.
A four way traffic light control system with count down timers is to be designed and constructed. The system is to be developed with the PIC16f877A chip being the microcontroller that is programmed to do the task of controlling. Figure shows the drawing of the 4-way junction, where each way has its traffic light and counter.
Low power LEDs are used for every traffic light with different colors, namely red, yellow and green. The red LED indicates “stop driving”, the yellow LED indicates “start stopping” and the green LED indicates “drive”. The sequence of altering the LEDs according to their color is as shown in the figure below: Green-Yellow-Red-Green. Twelve LEDs are used;three to each traffic light.
7-segment LED displays are used to show the current count value. Since all of the traffic lights are working simultaneously, each one is to display a different digit than the other. When a traffic light is tuned green, its corresponding 7-segment displays start counting down from a specific value and decrements until zero is reached. After this the counter starts by a new count value at the moment the yellow light turns on. When the red light turns on after the yellow took its time, the count continues to decrement until reaching zero. This means that the same 7-segments, on each traffic light, are used to display the count when cars are allowed and not allowed to pass. In terms of counting, the yellow and red are considered one set while the green is another set.
PDL (Program Description Language) :
- Turn on led_Red1
- Turn on led_Green2
- Wait 30 seconds
- Turn on led_Yellow1
- Turn on led_Yellow2
- Wait 3 seconds
- Turn on led_Red2
- Turn on led_Green1
- Wait 20 seconds
- Turn on led_Yellow1
- Turn on led_Yellow2
- Wait 3 seconds
Software Development
The code developed for the PIC is written in the C language. The compiler used to write the C code is mikroC PRO for PIC V. 6.6.3. After the c code is successfully compiled, a HEX file is generated.
Automatic traffic light using PIC16F877A Microcontroller (Code)
/*
* Project name:
Automatic traffic light control with microcontroller
* Copyright:
(c) lET'S THINk BINARY, 2017.
* Revision History:
V0.0
* Description:
Automatic traffic light using PIC Microcontroller .
* Test configuration:
MCU: PIC16f877a
Oscillator: 8.0000 MHz Crystal
SW: mikroC PRO for PIC
http://www.mikroe.com/mikroc/pic/
* NOTES:
- This Our Group (thanks to join and participate) :
https://www.facebook.com/groups/816450708460518/
- Facebook page (thanks to like and share) :
https://www.facebook.com/Lets-Think-Binary-1728910547340654/
- Youtube Channel (thanks to subscribe) :
https://www.youtube.com/channel/UCTIAzxEkyeA6HTvl_VHd-Tw
*/
#include "Display_Utils.h"
unsigned short shifter, portd_index;
unsigned short portd_array[4];
int digit,digit1, digit10,i;
sbit led_Green1 at portC.b0;
sbit led_Yellow1 at portC.b1;
sbit led_Red1 at portC.b2;
sbit led_Green2 at portC.b5;
sbit led_Yellow2 at portC.b6;
sbit led_Red2 at portC.b7;
void interrupt() {
PORTA = 0; // Turn off all 7seg displays
PORTD = portd_array[portd_index]; // bring appropriate value to PORTD
PORTA = shifter; // turn on appropriate 7seg. display
// move shifter to next digit
shifter <<= 1; if (shifter > 8u)
shifter = 1;
// increment portd_index
portd_index++ ;
if (portd_index > 3u)
portd_index = 0; // turn on 1st, turn off 2nd 7seg.
TMR0 = 0; // reset TIMER0 value
TMR0IF_bit = 0; // Clear TMR0IF
}
void display(){
digit = i % 10u;
digit1 = conversion(digit); // prepare ones digit
portd_array[0] = conversion(digit); // and store it to PORTD array
portd_array[2] = conversion(digit);
digit = (char)(i / 10u) % 10u;
digit10 = conversion(digit); // prepare tens digit
portd_array[1] = conversion(digit); // and store it to PORTD array
portd_array[3] = conversion(digit); // and store it to PORTD array
}
void main(){
ADCON1=6; // Configure PORTX pins as digital
TRISA = 0; // Configure PORTA as output
PORTA = 0; // Clear PORTA
TRISD = 0; // Configure PORTD as output
PORTD = 0; // Clear PORTD
TRISC=0;
PORTC=0;
OPTION_REG=0x80; // Timer0 settings
TMR0 = 0; // clear TMROL
digit = 0;
portd_index = 0;
shifter = 1;
GIE_bit = 1; // Enaable GIE
TMR0IE_bit = 1; // Enaable T0IE
//INTCON = 0xA0; // Enaable GIE,T0IE
do{
// PHASE1
PORTC=0;
i=30;
for(i;i>=0;i--){
display();
led_Green1=1; led_Red2=1;
delay_ms(400);
}
//PHASE2
PORTC=0;
i=3;
for(i;i>=0;i--){
display();
led_Yellow2=1;led_Yellow1=1;
delay_ms(400);
}
//PHASE3
PORTC=0;
i=20;
for(i;i>=0;i--){
display();
PORTC=0;
led_Green2=1; led_Red1=1;
delay_ms(400);
}
//PHASE4
PORTC=0;
i=3;
for(i;i>=0;i--){
display();
led_Yellow2=1;led_Yellow1=1;
delay_ms(400);
}
}while(1); // infinite loop
} // end of program
Automatic traffic light using PIC16F877A Microcontroller (Schematic Diagram)
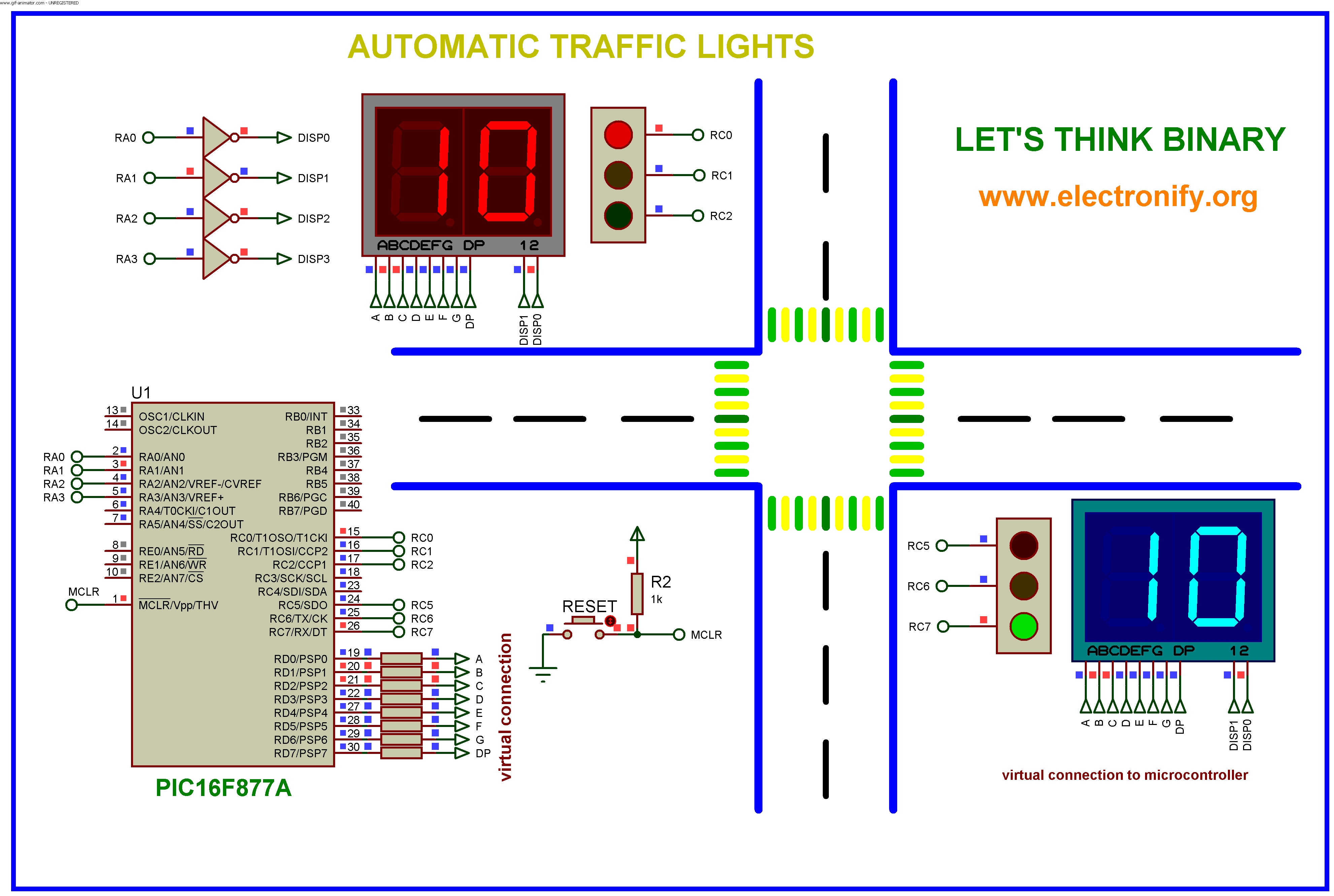
Results :
Compile the PIC code and get the hex file from it.
For simulating with PROTEUS ISIS hit run button and then you will get above output.
Resource :
You can download the MikroC Source Code and Proteus files etc from this link traffic light controller :
This Our Group (thanks to join and participate) : https://www.facebook.com/groups/816450708460518/
Facebook page : https://www.facebook.com/Lets-Think-Binary-1728910547340654/
Youtube Channel (thanks to subscribe) :
https://www.youtube.com/channel/UCTIAzxEkyeA6HTvl_VHd-Tw